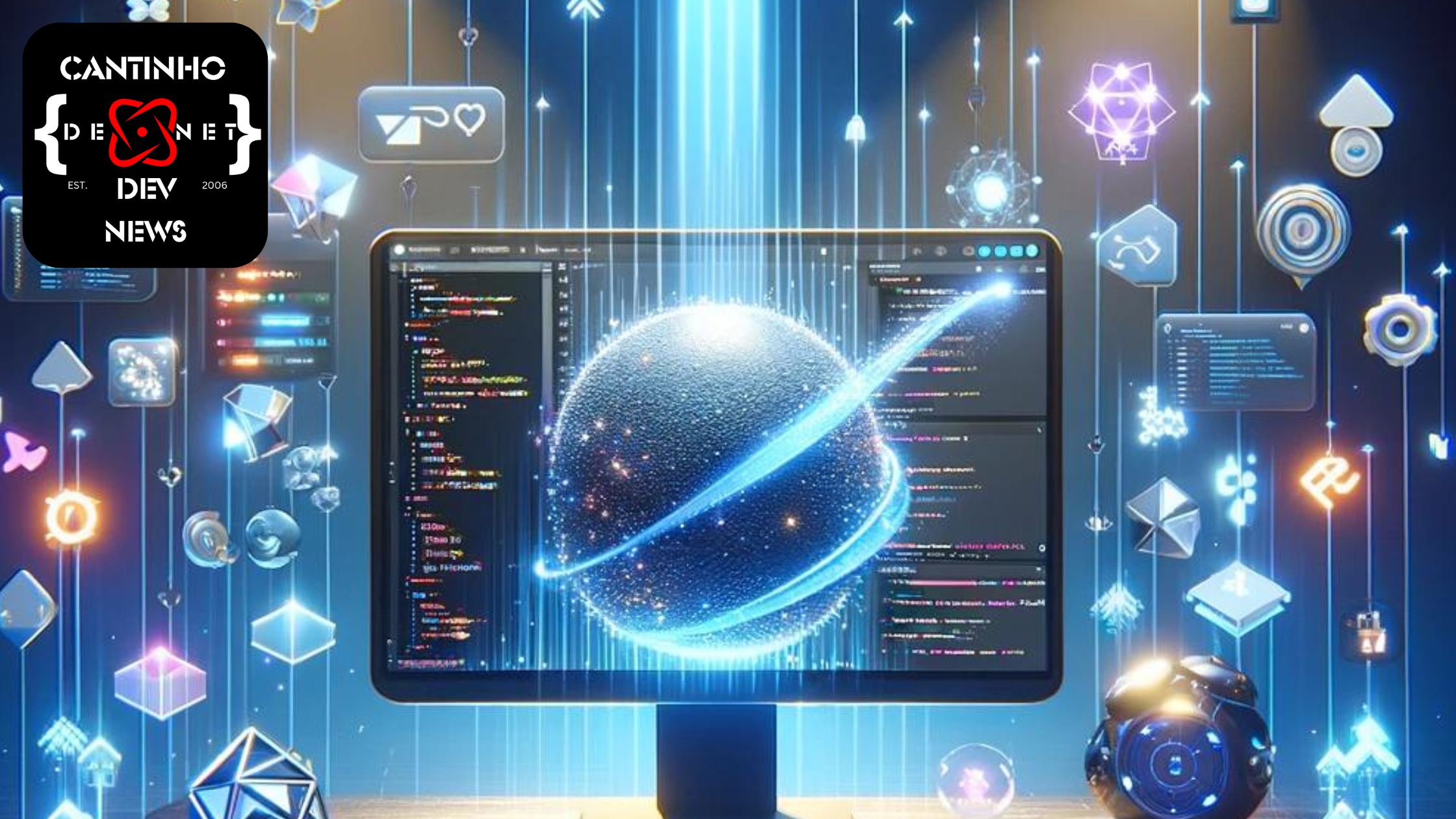
Workshop: Exploring Microservices Through Industry Examples
Running a Django site on shared hosting can be really agonizing. It’s budget-friendly, sure, but it comes with strings attached: sluggish response time and unexpected server hiccups. It kind of makes you want to give up.
Luckily, with a few fixes here and there, you can get your site running way smoother. It may not be perfect, but it gets the job done. Ready to level up your site? Let’s dive into these simple tricks that’ll make a huge difference.
Know Your Limits, Play Your Strengths
But before we dive deeper, let’s do a quick intro to Django. A website that is built on the Django web framework is called a Django-powered website.
Django is an open-source framework written in Python. It can easily handle spikes in traffic and large volumes of data. Platforms like Netflix, Spotify, and Instagram have a massive user base, and they have Django at their core.
Shared hosting is a popular choice among users when it comes to Django websites, mostly because it’s affordable and easy to set up. But since you’re sharing resources with other websites, you are likely to struggle with:
- Limited resources (CPU, storage, etc.)
- Noisy neighbor effect
However, that’s not the end of the world. You can achieve a smoother run by–
- Reducing server load
- Regular monitoring
- Contacting your hosting provider
These tricks help a lot, but shared hosting can only handle so much. If your site is still slow, it might be time to think about cheap dedicated hosting plans.
But before you start looking for a new hosting plan, let’s make sure your current setup doesn’t have any loose ends.
Flip the Debug Switch (Off!)
Once your Django site goes live, the first thing you should do is turn DEBUG
off. This setting shows detailed error texts and makes troubleshooting a lot easier.
This tip is helpful for web development, but it backfires during production because it can reveal sensitive information to anyone who notices an error.
To turn DEBUG
off, simply set it to False
in your settings.py
file.
DEBUG = False
Next, don’t forget to configure ALLOWED_HOSTS
. This setting controls which domains can access your Django site. Without it, your site might be vulnerable to unwanted traffic. Add your domain name to the list like this:
ALLOWED_HOSTS =['yourdomain.com', 'www.yourdomain.com']
With DEBUG
off and ALLOWED_HOSTS
locked down, your Django site is already more secure and efficient. But there’s one more trick that can take your performance to the next level.
Cache! Cache! Cache!
Imagine every time someone visits your site, Django processes the request and renders a response. What if you could save those results and serve them instantly instead? That’s where caching comes in.
Caching is like putting your site’s most frequently used data on the fast lane. You can use tools like Redis to keep your data in RAM. If it’s just about API responses or database query results, in-memory caching can prove to be a game changer for you.
To be more specific, there’s also Django’s built-in caching:
- Queryset caching: if your system is repeatedly running database queries, keep the query results.
- Template fragment caching: This feature caches the parts of your page that almost always remain the same (headers, sidebars, etc.) to avoid unnecessary rendering.
Optimize Your Queries
Your database is the backbone of your Django site. Django makes database interactions easy with its ORM (Object-Relational Mapping). But if you’re not careful, those queries can become a bone in your kebab.
- Use
.select_related()
and.prefetch_related()
When querying related objects, Django can make multiple database calls without you even realizing it. These can pile up and slow your site.
Instead of this:
posts = Post.objects.all()
for post in posts:
print(post.author.name) # Multiple queries for each post's author
Use this:
posts = Post.objects.select_related('author')
for post in posts:
print(post.author.name) # One query for all authors
- Avoid the N+1 Query Problem: The N+1 query problem happens when you unknowingly run one query for the initial data and an additional query for each related object. Always check your queries using tools like Django Debug Toolbar to spot and fix these inefficiencies.
- Index Your Database:
Indexes help your database find data faster. Identify frequently searched fields and ensure they’re indexed. In Django, you can add indexes like this:
class Post(models.Model):
title = models.CharField(max_length=200, db_index=True)
- Query Only What You Need:
Fetching unnecessary data wastes time and memory. Use.only()
or.values()
to retrieve only the fields you actually need.
Static Files? Offload and Relax
Static files (images, CSS, and JavaScript) can put a heavy load on your server. But have you ever thought of offloading them to a Content Delivery Network (CDN)? CDN is a dedicated storage service. The steps are as follows:
- Set Up a CDN (e.g., Cloudflare, AWS CloudFront):
A CDN will cache your static files and serve them from locations closest to your clients. - Use Dedicated Storage (e.g., AWS S3, Google Cloud Storage):
Store your files in a service designed for static content. Use Django’sstorages
library. - Compress and Optimize Files:
Minify your CSS and JavaScript files and compress images to reduce file sizes. Use tools likedjango-compressor
to automate this process.
By offloading static files, you’ll free up server storage and improve your site’s speed. It’s one more thing off your plate!
Lightweight Middleware, Heavyweight Impact
Middleware sits between your server and your application. It processes every request and response.
Check your MIDDLEWARE
setting and remove anything you don’t need. Use Django’s built-in middleware whenever you can because it’s faster and more reliable. If you create custom middleware, make sure it’s simple and only does what’s really necessary. Keeping middleware lightweight reduces server strain and uses fewer resources.
Frontend First Aid
Your frontend is the first thing users see, so a slow, clunky interface can leave a bad impression. Using your frontend the right way can dramatically improve the user experience.
-
Minimize HTTP Requests: Combine CSS and JavaScript files to reduce the number of requests.
-
Optimize Images: Use tools like TinyPNG or ImageOptim to compress images without losing quality.
-
Lazy Load Content: Delay loading images or videos until they’re needed on the screen.
-
Enable Gzip Compression: Compress files sent to the browser to reduce load times.
Monitor, Measure, Master
In the end, the key to maintaining a Django site is constant monitoring. By using tools like Django Debug Toolbar or Sentry, you can quickly identify performance issues.
Once you have a clear picture of what’s happening under the radar, measure your site’s performance. Use tools like New Relic or Google Lighthouse. These tools will help you prioritize where to make improvements. With this knowledge, you can optimize your code, tweak settings, and ensure your site runs smoothly.